Bài Tập Code C/C++ Cơ Bản Đến Nâng Cao Có Lời Giải - Phần 1
Có thể bạn quan tâm
- Home
- Lập Trình
- Bài Tập Code C/C++ Cơ Bản Đến Nâng Cao Có Lời Giải – Phần 1

Bài tập code C/C++ đơn giản dành cho các bạn vừa trải qua khóa học tổng quan về C/C++. Giúp các bạn củng cố và nắm chắc kiến thức hơn. Để chạy code các bạn có thể copy và vào trang này để chạy test nhá: https://www.onlinegdb.com/
Bài 1: Tính tổng S(n) = 1 + 2 + 3 + … + n
#include<stdio.h> #include<conio.h> int main() { int i, n; long S; S = 0; i = 1; printf("\nNhap n: "); scanf("%d", &n); while(i <= n) { S = S + i; i++; } printf("\nTong 1 + 2 + ... + %d la %ld: ", n, S); getch(); return 0; }Bài 2: Code C Tính tổng S(n) = 1^2 + 2^2 + … + n^2
#include<stdio.h> #include<conio.h> int main() { int i, n; long S; S = 0; i = 1; printf("\nNhap n: "); scanf("%d", &n); while(i <= n) { S = S + i * i; i++; } printf("i = %d", i); printf("\nTong 1^2 + 2^2 + ... + %d^2 la: %ld", n, S); getch(); return 0; }Bài 3: Tính tổng S(n) = 1 + ½ + 1/3 + … + 1/n
#include<stdio.h> #include<conio.h> int main() { int i, n; float S; S = 0; i = 1; do { printf("\nNhap n: "); scanf("%d", &n); if(n < 1) { printf("\nN phai lon hon hoac bang 1. Xin nhap lai !"); } }while(n < 1); while(i <= n) { S = S + 1.0 / i; // phải nhớ là 1.0 / i i++; } printf("i = %d", i); printf("\nS = %f", S); printf("\nTong 1 + 1/2 + ... + 1/%d la %.2f: ",n, S); getch(); return 0; }Bài 4: Tính tổng S(n) = ½ + ¼ + … + 1/2n
#include<stdio.h> #include<conio.h> int main() { int i, n; float S; S = 0; do { printf("\nNhap n: "); scanf("%d", &n); if(n < 1) { printf("\nN phai lon hon hoac bang 1. Xin nhap lai !"); } }while(n < 1); for(i = 1; i <= n; i++) { S = S + 1.0 / (2 * i); } printf("\nTong la: %f", S); getch(); return 0; }Bài 5: Tính tổng S(n) = 1 + 1/3 + 1/5 + … + 1/(2n + 1)
#include<stdio.h> #include<conio.h> int main() { int i, n; float S; S = 0; do { printf("\nNhap n: "); scanf("%d", &n); if(n < 1) { printf("\nN phai lon hon hoac bang 1. Xin nhap lai !"); } }while(n < 1); for(i = 0; i < n; i++) { S = S + 1.0 / ((2 * i) + 1); } printf("\nTong la: %f", S); getch(); return 0; }Bài 6: Tính tổng S(n) = 1/1×2 + 1/2×3 +…+ 1/n x (n + 1)
#include<stdio.h> #include<conio.h> int main() { int i, n; float S; S = 0; i = 1; do { printf("\nNhap n: "); scanf("%d", &n); if(n < 1) { printf("\nN phai lon hon hoac bang 1. Xin nhap lai !"); } }while(n < 1); while(i <= n) { S = S + 1.0 / (i * (i + 1)); i++; } printf("\nTong la %f", S); getch(); return 0; }Bài 7: Tính tổng S(n) = ½ + 2/3 + ¾ + …. + n / n + 1
#include<stdio.h> #include<conio.h> int main() { int i, n; float S; S = 0; i = 1; do { printf("\nNhap n: "); scanf("%d", &n); if(n < 1) { printf("\nN phai lon hon hoac bang 1. Xin nhap lai !"); } }while(n < 1); while(i <= n) { S = S + (float)i / (i + 1); i++; } printf("\nTong la %f", S); getch(); return 0; }Bài 8: Tính tổng S(n) = ½ + ¾ + 5/6 + … + 2n + 1/ 2n + 2
#include<stdio.h> #include<conio.h> int main() { int i, n; float S; S = 0; i = 0; do { printf("\nNhap n: "); scanf("%d", &n); if(n < 1) { printf("\nN phai lon hon hoac bang 1. Xin nhap lai !"); } }while(n < 1); while(i < n) { S = S + (float)(2 * i + 1) / (2 * i + 2); i++; } printf("\nTong la %f", S); getch(); return 0; }Bài 9: Tính tổng T(n) = 1 x 2 x 3…x N
#include<stdio.h> #include<conio.h> int main() { int i, n; long P; i = 1; P = 1; do { printf("\nNhap n: "); scanf("%d", &n); if(n < 1) { printf("\nN phai lon hon hoac bang 1. Xin nhap lai !"); } }while(n < 1); while(i < n) { P = P * i; i++; } printf("\nTich 1x2x....x%d la: %ld", i, P); getch(); return 0; }Bài 10: Tính tổng T(x, n) = x^n
#include<stdio.h> #include<conio.h> double Power_n(double x, long n) { // n >= 0 double result = 1; while(n--) { result = result * x; } return result; } double qPower_n(double x, long n) { // n >= 0 double result = 1; while(n) { if(n % 2 == 1) { result = result * x; } x = x * x; n = n / 2; } return result; } int main() { double x = 3; long n = 2; double z; z = qPower_n(x, n); printf("z = %f", z); getch(); return 0; }Bài 11: Tính tổng S(n) = 1 + 1.2 + 1.2.3 + … + 1.2.3….N
#include<stdio.h> #include<conio.h> int main() { int i, n; long S, P; S = 0; P = 1; i = 1; do { printf("\nNhap n: "); scanf("%d", &n); if(n < 1) { printf("\nN phai lon hon hoac bang 1. Xin nhap lai !"); } }while(n < 1); while(i <= n) { P = P * i; S = S + P; i++; } printf("\nTong la %d",S); getch(); return 0; }Bài 12: Tính tổng S(n) = x + x^2 + x^3 + … + x^n
#include<stdio.h> #include<conio.h> int main() { int i, n; float x, T, S; i = 1; T = 1; S = 0; printf("\nNhap x: "); scanf("%f", &x); printf("\nNhap n: "); scanf("%d", &n); while(i <= n) { T = T * x; S = S + T; i++; } printf("\nTong la %f",S); getch(); return 0; }Bài 13: Tính tổng S(n) = x^2 + x^4 + … + x^2n
#include<stdio.h> #include<conio.h> #include<math.h> int main() { int i, n; float x, T, S; i = 1; T = 1; S = 0; printf("\nNhap x: "); scanf("%f", &x); printf("\nNhap n: "); scanf("%d", &n); while(i <= n) { //T = T * x * x; T = pow(x, (2 * i )); S = S + T; i++; } printf("\nTong la %f",S); getch(); return 0; }Bài 14: Tính tổng S(n) = x + x^3 + x^5 + … + x^2n + 1
#include<stdio.h> #include<conio.h> #include<math.h> int main() { int i, n; float x, T, S; i = 0; T = 1; S = 0; printf("\nNhap x: "); scanf("%f", &x); printf("\nNhap n: "); scanf("%d", &n); while(i < n) { T = pow(x, (2 * i + 1)); S = S + T; i++; } printf("\nTong la %f",S); getch(); return 0; }Bài 15: Tính tổng S(n) = 1 + 1/1 + 2 + 1/ 1 + 2 + 3 + ….. + 1/ 1 + 2 + 3 + …. + N
#include<stdio.h> #include<conio.h> int main() { int i, n; float S, T; i = 1; S = 0; T = 0; do { printf("\nNhap n: "); scanf("%d", &n); if(n < 1) { printf("\nN phai >= 1. Xin Nhap lai!"); } }while(n < 1); while(i <= n) { T = T + i; S = S + 1.0 / T; i++; } printf("\nTong la %f", S); getch(); return 0; }Bài 16: Code C Tính tổng S(n) = x + x^2/1 + 2 + x^3/1 + 2 + 3 + … + x^n/1 + 2 + 3 + …. + N
#include<stdio.h> #include<conio.h> int main() { int i, n; float x, S, T; long M; printf("\nNhap x: "); scanf("%f", &x); do { printf("\nNhap n: "); scanf("%d", &n); if(n < 1) { printf("\n N phai >= 1. Xin nhap lai !"); } }while(n < 1); S = 0; T = 1; M = 0; i = 1; while(i <= n) { T = T * x; M = M + i; S = S + T/M; i++; } printf("\nTong la %f", S); getch(); return 0; }Bài 17: Code C Tính tổng S(n) = x + x^2/2! + x^3/3! + … + x^n/N!
#include<stdio.h> #include<conio.h> int main() { int i, n; float x, S, T; long M; printf("\nNhap x: "); scanf("%f", &x); do { printf("\nNhap n: "); scanf("%d", &n); if(n < 1) { printf("\n N phai >= 1. Xin nhap lai !"); } }while(n < 1); S = 0; T = 1; M = 1; i = 1; while(i <= n) { T = T * x; M = M * i; S = S + T/M; i++; } printf("\nTong la %f", S); getch(); return 0; }Bài 18: Code C Tính tổng S(n) = 1 + x^2/2! + x^4/4! + … + x^2n/(2n)!
#include<stdio.h> #include<conio.h> #include<math.h> int main() { int i, n; float x, S, T; long M, N; printf("\nNhap x: "); scanf("%f", &x); do { printf("\nNhap n(n >= 0) : "); scanf("%d", &n); if(n < 0) { printf("\n N phai >= 0. Xin nhap lai !"); } }while(n < 0); S = 1; N = 1; i = 1; while(i <= n) { T = pow(x, (2 * i )); M = i * 2; N = N * M * (M - 1); S = S + T/N; i++; } printf("\nTong la %f", S); getch(); return 0; }Bài 19: Tính tổng S(n) = 1 + x + x^3/3! + x^5/5! + … + x^(2n+1)/(2n+1)!
#include<stdio.h> #include<conio.h> #include<math.h> int main() { int i, n; float x, S, T; long M, N; printf("\nNhap x: "); scanf("%f", &x); do { printf("\nNhap n(n >= 1) : "); scanf("%d", &n); if(n < 1) { printf("\n N phai >= 1. Xin nhap lai !"); } }while(n < 1); S = 1; N = 1; i = 1; while(i <= n) { T = pow(x, (2 * i + 1)); M = i * 2 + 1; N = N * M * (M - 1); S = S + x + T/N; i++; } printf("\nTong la %f", S); getch(); return 0; }Bài 20: Liệt kê tất cả các ước số của số nguyên dương n
#include<stdio.h> #include<conio.h> int main() { int i, n; do { printf("\nNhap n(n > 0): "); scanf("%d", &n); if(n <= 0) { printf("\n N phai > 0. Xin nhap lai !"); } }while(n <= 0); i = 1; while(i <= n) { if(n % i == 0) printf("%4d", i); i++; } getch(); return 0; }Bài 21: Tính tổng tất cả các ước số của số nguyên dương n
#include<stdio.h> #include<conio.h> int main() { int i, n; long S; do { printf("\nNhap n(n > 0): "); scanf("%d", &n); if(n <= 0) { printf("\n N phai > 0. Xin nhap lai !"); } }while(n <= 0); i = 1; S = 0; while(i <= n) { if(n % i == 0) { printf("%4d", i); S = S + i; } i++; } printf("\nTong cac uoc cua %d la: %ld", n, S); getch(); return 0; }Bài 22: Tính tích tất cả các ước số của số nguyên dương n
#include<stdio.h> #include<conio.h> int main() { int i, n; long P; do { printf("\nNhap n(n > 0): "); scanf("%d", &n); if(n <= 0) { printf("\n N phai > 0. Xin nhap lai !"); } }while(n <= 0); i = 1; P = 1; while(i <= n) { if(n % i == 0) { printf("%4d", i); P = P * i; } i++; } printf("\nTich cac uoc cua %d la: %ld", n, P); getch(); return 0; }Bài 23: Đếm số lượng ước số của số nguyên dương n
#include<stdio.h> #include<conio.h> int main() { int i, n; int count; do { printf("\nNhap n(n > 0): "); scanf("%d", &n); if(n <= 0) { printf("\n N phai > 0. Xin nhap lai !"); } }while(n <= 0); i = 1; count = 0; while(i <= n) { if(n % i == 0) { printf("%4d", i); count++; } i++; } printf("\nSo luong cac uoc so cua %d la: %d", n , count); getch(); return 0; }Bài 24: Liệt kê tất cả các ước số lẻ của số nguyên dương n
#include<stdio.h> #include<conio.h> int main() { int i, n; do { printf("\nNhap n(n > 0): "); scanf("%d", &n); if(n <= 0) { printf("\n N phai > 0. Xin nhap lai !"); } }while(n <= 0); i = 1; printf("\nCac uoc so le cua so %d la: ",n); while(i <= n) { if(n % i == 0) { if(i % 2 == 1) printf("%4d", i); } i++; } getch(); return 0; }Bài 25: Tính tổng tất cả các ước số chẵn của số nguyên dương n
#include<stdio.h> #include<conio.h> int main() { int i, n; long S; do { printf("\nNhap n(n > 0): "); scanf("%d", &n); if(n <= 0) { printf("\n N phai > 0. Xin nhap lai !"); } }while(n <= 0); i = 1; S = 0; printf("\nCac uoc so chan cua so %d la: ",n); while(i <= n) { if(n % i == 0) { if(i % 2 == 0) { printf("%4d", i); S = S + i; } } i++; } printf("\nTong cac uoc so chan cua %d la: %ld", n, S); getch(); return 0; }Bài 26: Tính tích tất cả các ước số lẻ của số nguyên dương n
#include<stdio.h> #include<conio.h> int main() { int i, n; long P; do { printf("\nNhap n(n > 0): "); scanf("%d", &n); if(n <= 0) { printf("\n N phai > 0. Xin nhap lai !"); } }while(n <= 0); i = 1; P = 1; printf("\nCac uoc so le cua so %d la: ",n); while(i <= n) { if(n % i == 0) { if(i % 2 == 1) { printf("%4d", i); P = P * i; } } i++; } printf("\nTich cac uoc so le cua %d la: %ld", n, P); getch(); return 0; }Bài 27: Đếm số lượng ước số chẵn của số nguyên dương n
#include<stdio.h> #include<conio.h> int main() { int i, n; int count; do { printf("\nNhap n(n > 0): "); scanf("%d", &n); if(n <= 0) { printf("\n N phai > 0. Xin nhap lai !"); } }while(n <= 0); i = 1; count = 0; printf("\nCac uoc so chan cua so %d la: ",n); while(i <= n) { if(n % i == 0) { if(i % 2 == 0) { printf("%4d", i); count++; } } i++; } printf("\nSo luong uoc so chan cua %d la: %ld", n, count); getch(); return 0; }Bài 28: Cho số nguyên dương n. Tính tổng các ước số nhỏ hơn chính nó. ( Tính tổng các ước số nhỏ hơn n )
#include<stdio.h> #include<conio.h> int main() { int i, n; long S; do { printf("\nNhap n(n > 0): "); scanf("%d", &n); if(n <= 0) { printf("\n N phai > 0. Xin nhap lai !"); } }while(n <= 0); i = 1; S = 0; printf("\nCac uoc nho hon %d la",n); while(i < n) { if(n % i == 0) { printf("%4d", i); S = S + i; } i++; } printf("\nTong cac uoc nho hon %d la: %ld", n, S); getch(); return 0; }Bài 29: Tìm ước số lẻ lớn nhất của số nguyên dương n
#include<stdio.h> #include<conio.h> int main() { int i, n, max; do { printf("\nNhap n(n > 0): "); scanf("%d", &n); if(n <= 0) { printf("\n N phai > 0. Xin nhap lai !"); } }while(n <= 0); i = 1; max = 1; printf("\nCac uoc so le cua so %d la: ",n); while(i <= n) { if((n % i == 0) && (i % 2 == 1)) { if(i > max) { max = i; } printf("%4d", i); } i++; } printf("\nUoc so le lon nhat la %d", max); getch(); return 0; }Bài 30: Kiểm tra số nguyên dương n có phải là số hoàn thiện hay không?
#include<stdio.h> #include<conio.h> int main() { int i, n; long S; do { printf("\nNhap n(n > 0): "); scanf("%d", &n); if(n <= 0) { printf("\n N phai > 0. Xin nhap lai !"); } }while(n <= 0); i = 1; S = 0; while(i < n) { if(n % i == 0) { S = S + i; } i++; } if(S == n) printf("\n%d la so hoan thien", n); else printf("\nSo nhap vao khong la so hoan thien"); getch(); return 0; }Bài 31: Kiểm tra số nguyên dương n có phải là số nguyên tố hay không?
#include<stdio.h> #include<conio.h> int main() { int i, n; printf("\nNhap n: "); scanf("%d", &n); if(n < 2) printf("\nSo %d khong phai la so nguyen to", n); else if(n == 2) printf("\nSo %d la so nguyen to", n); else if(n % 2 == 0) printf("\nSo %d khong phai la so nguyen to", n); else { for(i = 3; i <= n; i+=2) { if(n % i == 0) break; } if(i == n) printf("\nSo %d la so nguyen to", n); else printf("\nSo %d khong phai la so nguyen to", n); } getch(); return 0; }Bài 32: Kiểm tra số nguyên dương n có phải là số chính phương hay không?
#include<stdio.h> #include<conio.h> #include<math.h> int main() { int i, n; do { printf("\nNhap n: "); scanf("%d", &n); if(n <= 0) printf("\nn phai > 0. Xin nhap lai !"); }while(n <= 0); /*Số chính phương là số mà kết quả khai căn bậc 2 là 1 số nguyên sqrt(4) = 2.00000 => ép về nguyên = 2 => vì 2.000 == 2 (true) => là số chính phương sqrt(5) = 2.23234 => ép về nguyên = 2 => vì 2.4324 != 2 (false) => không là số chính phương*/ if (sqrt((float)n) == (int)sqrt((float)n)) // So sánh 2 số khi chưa ép về kiểu nguyên và số đã ép về kiểu nguyên { printf("\n%d La so chinh phuong", n); } else { printf("\n%d Khong la so chinh phuong", n); } getch(); return 0; }Bài 33: Cho n là số nguyên dương. Hãy tìm giá trị nguyên dương k lớn nhất sao cho S(k) < n. Trong đó chuỗi k được định nghĩa như sau: S(k) = 1 + 2 + 3 + … + k
#include<stdio.h> int main(){ int i; int n; int s=0; do { printf("Nhap vap n:\n"); scanf("%d",&n); if(n < 1) { printf("n phai lon hon bang 1\n"); } }while(n < 1); for(i=1;i<=n;i++) { s = s + i ; if(s>=n) { printf("So k can tim la : %d",(i-1)); break; } } return 0; }Cảm ơn các bạn đã ghé thăm. Chúc các bạn thành công!
code c/c++ 180 lượt xemMạng xã hội
Hoặc copy link
Copy 0 Báo lỗi Chia sẻ Đề xuất cho bạn
Get all comments Facebook
6 tháng trước
Multiple Databases in Spring Boot / JdbcTemplate
12 tháng trước
How to css Gallery image JS
1 năm trước
How to Fix Error Connection refused no further information
1 năm trước
Get IP Address Client In Java
1 năm trước
Get IP Address Client In Reactjs
1 năm trước
Get IP Address Server In Java
1 năm trước
Fix Error The error code 0x5 5 occurred during initialization
1 năm trướcKyojuro Rengoku Hitman Anime Wallpaper Video
Khi nào nên dùng WebClient Spring Boot
Lofi Studio Live Video Wallpaper 4k
MỚI XEM NỔI
Anime Wallpaper Video Byakuya Kuchiki Bleach 4K
Download Video
Anime Wallpaper Video Inosuke Kimetsu No Yaiba 3440×1440
Download Video
Wallpaper Video Epic Moon and Clouds 4K
Download Video
Đồ án Python Code Game Racing ( Full Báo cáo + Slide PP )
Đồ Án Python
Ram Re Zero 4K Anime Wallpaper Video
Download Video
Get all comments Facebook
Code Hay Lập Trình Tin tức
Báo Cáo Quản Trị Dự Án Xây dựng hệ thống quản lý nhà hàng
Công nghệ thông tin Tài Liệu
Báo Cáo Quản Trị Dự Án Xây dựng hệ thống quản lý kho hàng
Công nghệ thông tin Tài Liệu
Download Video Wallpaper Agatsuma Zenitsu – Anime Kimetsu No Yaiba
Download Video
500 Câu Trắc Nghiệm Mạng Máy Tính Phần 1 Có Đáp Án
Lập Trình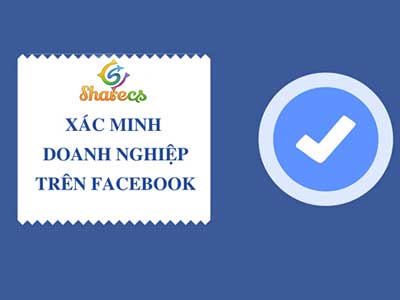
Fake Giấy Tờ Xác Minh Doanh Nghiệp Trên Facebook
Thủ thuật internet
File Mẫu Test Case Excel Chuẩn Nhất
Lập Trình Thủ thuật phần mềm
500 Câu Trắc Nghiệm Mạng Máy Tính Phần 2 Có Đáp Án
Lập Trình
Dark Nights Sachiro Anime Wallpaper Video
Download Video
Nhận Diện Chó Mèo Python – Tensorflow – Neural Network – Deep Learning
Python
Trắc Nghiệm Mạng Máy Tính Phần 3 Có Đáp Án
Lập Trình
Anime Wallpaper Video Emilia Nikke 4K
Download Video
Tool Active Win 10 Pro Và Active Office
Thủ Thuật Thủ thuật máy tính Thủ thuật phần mềm Windows
Anime Wallpaper Video Byakuya Kuchiki Bleach 4K
Download Video
Anime Wallpaper Fate Grand Order Video 4k
Download Video
Anime Wallpaper Kirito Asuna Sword Art Online Video 4k
Download Video
Download Video Wallpaper Code Is Life
Download Video
Wallpaper Video 4k Abstract – Room
Download Video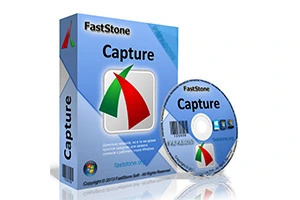
Hướng dẫn sử dụng FastStone Capture 2024
Thủ Thuật Thủ thuật phần mềmTừ khóa » Code C Có Lời Giải
-
Bài Tập C Có Lời Giải - Học Lập Trình C Online - Viettuts
-
1000 Bài Tập Lập Trình C/C++ Có Lời Giải Của Thầy Khang
-
Tổng Hợp Bài Tập C/C++ Có Lời Giải - Lập Trình Không Khó
-
Tổng Hợp Hơn 1000 Bài Tập C / C++ Có Lời Giải - Freetuts
-
Tuyển Tập 140 Bài Tập C Có Giải
-
Bài Tập C++ Có Lời Giải (code Mẫu)
-
1000 Bài Tập Lập Trình C/C++ Có Lời Giải Của Thầy Khang PDF
-
Bai Tap C++ Co Ban Co Loi Giai? - Tạo Website
-
Bài Tập Ngôn Ngữ Lập Trình C Có Lời Giải - 123doc
-
Bài Tập C++ Có Lời Giải? - Sapphire
-
Trọn Bộ Bài Tập Lập Trình Hướng đối Tượng Có Lời Giải Và đề || Code C ...
-
Bài Tập C++ Có Lời Giải/Nhập Xuất Dữ Liệu - Wikibooks
-
Bài Tập C++ Có Lời Giải/Một Số Bài Tập Nâng Cao - Wikibooks
-
Download Bài Tập C Và C++ Có Lời Giải -Tải Về Mới Nhất