What Are 0x01 And 0x80 Representative Of In C Bitwise Operations?
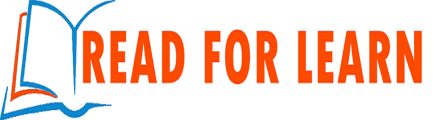
0x01 is the least significant bit set, hence the decimal value is 1.
0x80 is the most significant bit of an 8-bit byte set. If it is stored in a signed char (on a machine that uses 2’s-complement notation – as most machines you are likely to come across will), it is the most negative value (decimal -128); in an unsigned char, it is decimal +128.
The other pattern that becomes second nature is 0xFF with all bits set; this is decimal -1 for signed characters and 255 for unsigned characters. And, of course, there’s 0x00 or zero with no bits set.
What the loop does on the first cycle is to check if the LSB (least significant bit) is set, and if it is, sets the MSB (most significant bit) in the result. On the next cycle, it checks the next to LSB and sets the next to MSB, etc.
| MSB | | | | | | | LSB | | 1 | 0 | 1 | 1 | 0 | 0 | 1 | 1 | Input | 1 | 1 | 0 | 0 | 1 | 1 | 0 | 1 | Output | 1 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0x80 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0x01 | 0 | 1 | 0 | 0 | 0 | 0 | 0 | 0 | (0x80 >> 1) | 0 | 0 | 0 | 0 | 0 | 0 | 1 | 0 | (0x01 << 1)Related Posts:
- When a number is written as 0x00… what does the x mean
- waitpid, wnohang, wuntraced. How do I use these
- How to do scanf for single char in C
- Break statement not within loop or switch in C
- How to properly malloc for array of struct in C
- Cache Simulator in C
- C: error: expected ‘)’ before ‘;’ token
- Where is the C auto keyword used?
- execv vs execvp, why just one of them require the exact file’s path?
- difference between and
- expected expression before ‘{‘ token
- C Vector/ArrayList/LinkedList
- Convert char array to a int number in C
- Bind failed: Address already in use
- Warning/error “function declaration isn’t a prototype”
- What is the difference between %f and %lf in C?
- Returning an array using C
- What is stdin in C language?
- How to prevent multiple definitions in C?
- How to pass 2D array (matrix) in a function in C?
- How can you print multiple variables inside a string using printf?
- C dynamically growing array
- Why should we check WIFEXITED after wait in order to kill child processes in Linux system call?
- How to compile makefile using MinGW?
- Mapping a numeric range onto another
- Why are hexadecimal numbers prefixed with 0x?
- Portable way to check if directory exists [Windows/Linux, C]
- too many arguments for format [-Wformat-extra-args]
- How to copy a char array in C?
- Simple way to check if a string contains another string in C?
- cast to pointer from integer of different size, pthread code
- Invalid type argument of unary ‘*’ (have ‘int’) Error in C
- Why do I get “cast from pointer to integer of different size” error?
- C subscripted value is neither array nor pointer nor vector when assigning an array element value
- error: too few arguments to function `printDay’ (C language)
- How to convert integer to char in C?
- Where to find the complete definition of off_t type?
- struct has no member named
- printf not printing to screen
- Initializing array of structures
- Still Reachable Leak detected by Valgrind
- Assembly x86 – “leave” Instruction
- What is the difference between array and enum in C ?
- What is signed integer overflow?
- When to use const char * and when to use const char []
- What do numbers using 0x notation mean?
- Can I create an Array of Char pointers in C?
- Does stack grow upward or downward?
- Convert Little Endian to Big Endian
- Excess elements of scalar initializer for pointer to array of ints
- error C2371: ‘functionname’ redefinition: different basic types
- How does one represent the empty char?
- How to write to a file using open() and printf()?
- note: previous implicit declaration of ‘point_forward’ was here
- Can I define a function inside a C structure?
- Where is the header file on Linux? Why can’t I find ?
- What exactly is meant by “de-referencing a NULL pointer”?
- Difference between fgets and fscanf?
- Why is the sizeof(int) == sizeof(long)?
- getc() vs fgetc() – What are the major differences?
- Can’t understand the working of getint() in C as per K&R
- Tokenizing strings in C
- How to allocate array of pointers for strings by malloc in C?
- Realloc Invalid Pointer in C
- What tools are there for functional programming in C?
- typedef fixed length array
- How to format strings using printf() to get equal length in the output
- In C, what exactly happens when you pass a NULL pointer to strcmp()?
- “-bash: gcc: command not found” using cygwin when compiling c?
- How to format strings using printf() to get equal length in the output
- lseek/write suddenly returns -1 with errno = 9 (Bad file descriptor)
- Should I use printf(“\n”) or putchar(‘\n’) to print a newline in C?
- What do \t and \b do?
- Why and when to use static structures in C programming?
- error: struct has no member named X
- Where does linux store my syslog?
- Example of waitpid() in use?
- Can a function return two values?
- C: warning: excess elements in array initializer; near initialization for ‘xxx’ ; expects ‘char *’, but has type ‘int’
- write() to stdout and printf output not interleaved?
- How to know what the ‘errno’ means?
- Split string with multiple delimiters using strtok in C
- Printf was not declared in this scope
- warning: missing terminating ” character [enabled by default]
- How to free memory from char array in C
- Can I get Unix’s pthread.h to compile in Windows?
- Initializing 2D char array in C
- FFT in a single C-file
- Process exited with return value 3221225477
- How do I check if a string contains a certain character?
- Using pointer to char array, values in that array can be accessed?
- How can I create a dynamically sized array of structs?
- malloc(sizeof(int)) vs malloc(sizeof(int *)) vs (int *)malloc(sizeof(int))
- %p Format specifier in c
- Level vs Edge Trigger Network Event Mechanisms
- “Nothing to be done for makefile” message
- Convert Char to String in C
- How to use shared memory with Linux in C
- When is it ok to use a global variable in C?
- What is the difference between signed and unsigned int
Leave a Comment Cancel reply
Comment
Name Email Website Search for:Recommended Hostings
Cloudways: Realize Your Website's Potential With Flexible & Affordable Hosting. 24/7/365 Support, Managed Security, Automated Backups, and 24/7 Real-time Monitoring.
FastComet: Fast SSD Hosting, Free Migration, Hack-Free Security, 24/7 Super Fast Support, 45 Day Money Back Guarantee.
Recent Added Topics
- Can’t access wordpress mgt dashboard until propogation finished?
- “The Link You Followed Has Expired” error when saving a post (randomly)
- Multimedia upload error in my wordpress job board plugin
- How to assign to each output values from foreach loop to a meta_key?
- How do I access the current post object within a block theme template or pattern?
- Installation échouée : Le téléchargement a échoué. No working transports found
- Woocommerce deleted and replaced my WordPress front/page. How do I restore my original page?
- Display search results within same page using elementor as page builder
- Send and receive emails with wp_mail() for WP User (like Mailserver)
- Remove 3rd party plugin notices from within own plugin
Từ khóa » C 0x80
-
What Is Char I=0x80 And Why Overflow Did Not Happen In Bit Shifting
-
C Programming :: Bitwise Operators - Discussion - IndiaBIX
-
What Are 0x01 And 0x80 Representative Of In C Bitwise ... - Newbedev
-
What Are 0x01 And 0x80 Representative Of In C ... - CopyProgramming
-
Cx51 User's Guide: Sfr - Keil
-
WERA 05030116001 Micro Screwdriver PZ 4,0x80 Mm - Reichelt
-
Manufactured By AMETEK DUNKERMOTOREN
-
Pre-owned - EBay
-
0x80 Zadań Z C I C++ | Request PDF - ResearchGate
-
'utf-8' Codec Can't Decode Byte 0x80 In Position 65: Invalid ... - GitHub
-
Linux Exit System Call By Int 0x80 - Gists · GitHub
-
9008320000 SDS 0.5X3.0X80 | Weidmüller Product Catalogue
-
Q39311: Use 0x80 To Access Drive C When Calling _bios_disk
-
At Drivers/scsi/bfa/bfa_fcpim.c:2629 Bfa_ioim_good_comp_isr+0x77 ...